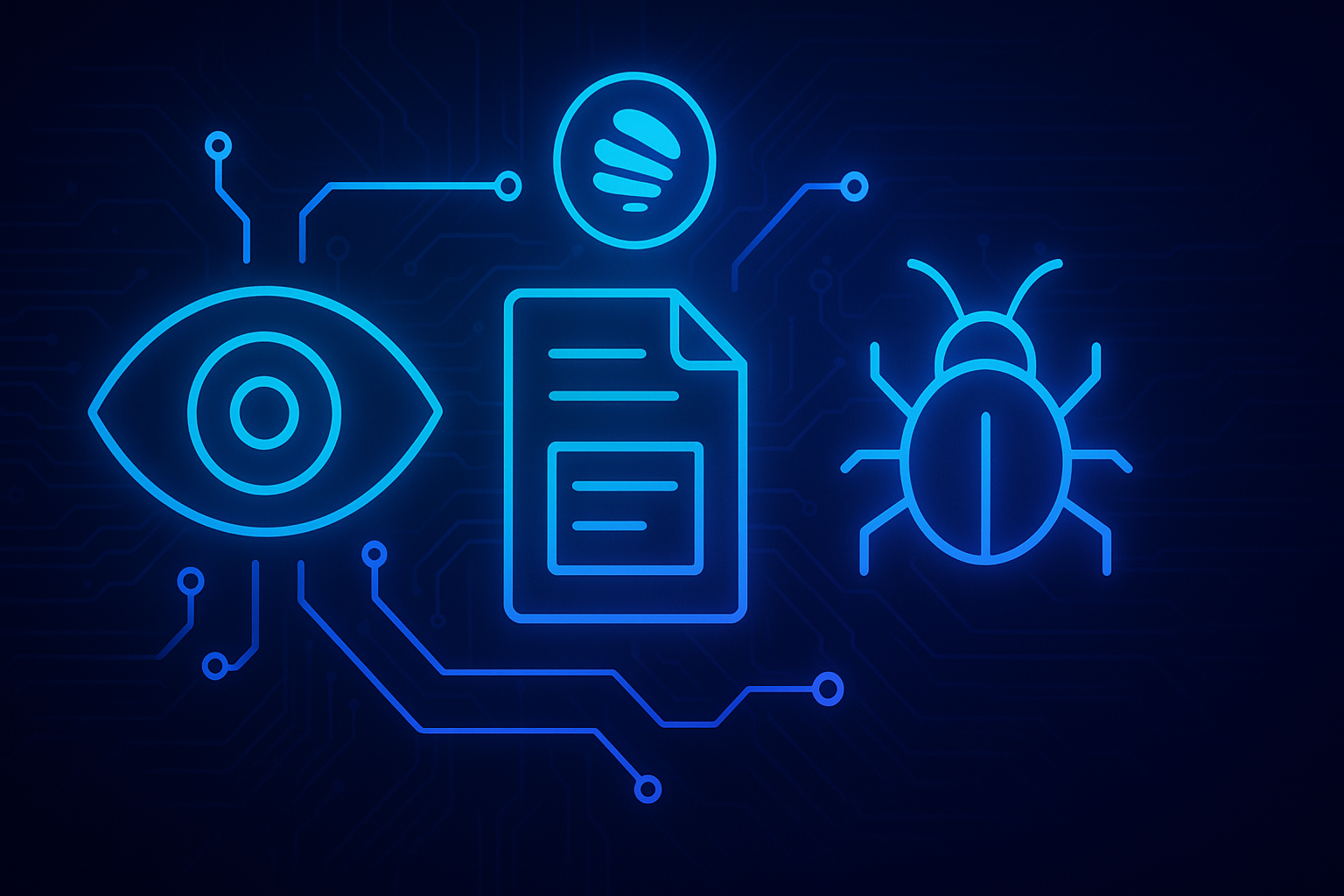
Integrating Sentry in .NET MAUI with Local File Logging
Sentry is a powerful tool for error tracking and diagnostics, and in my TwistReader MAUI app, I wanted to go beyond just capturing errors in production. The goal: use Sentry for all logging, but pe...