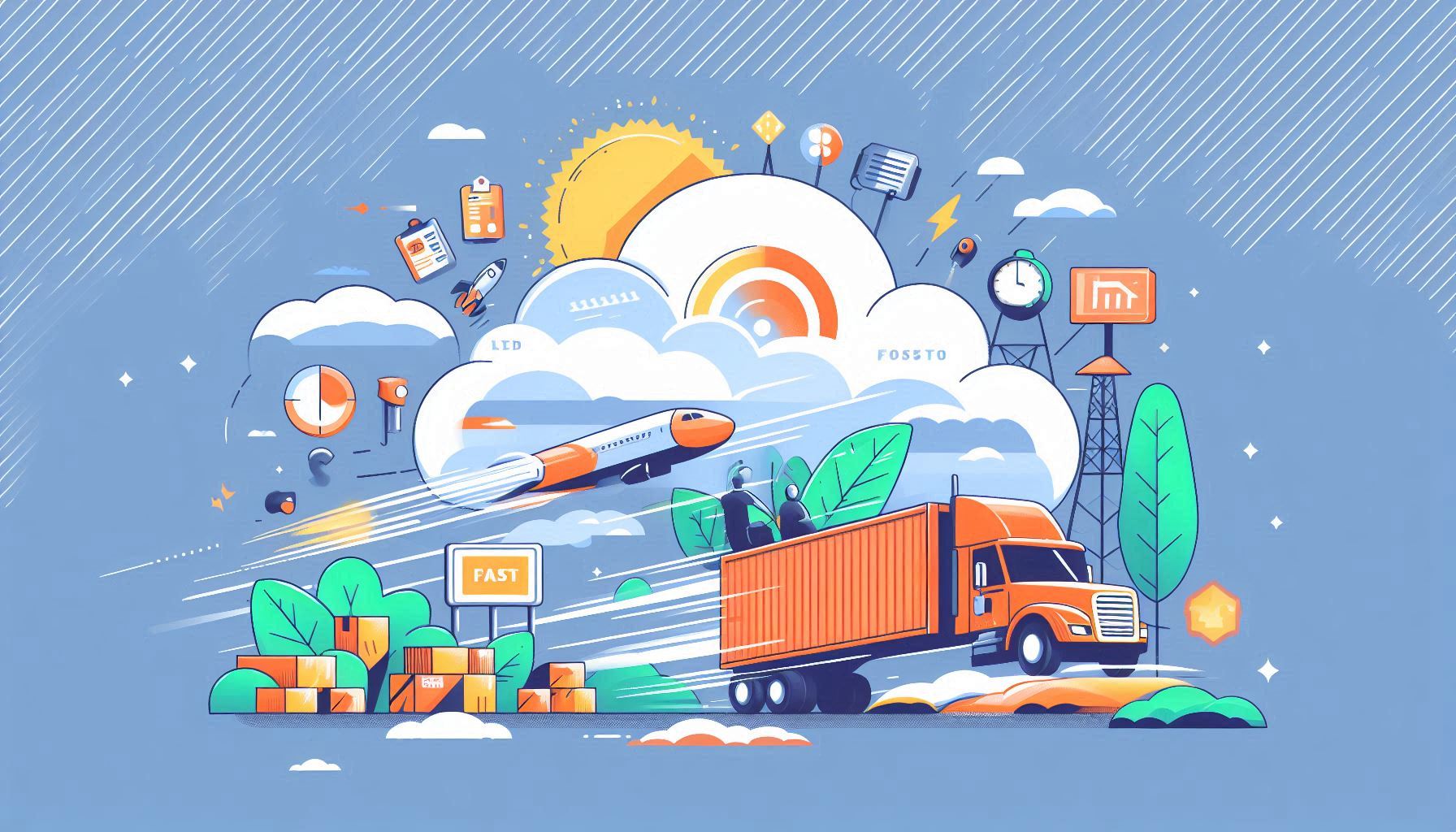
Welcome from the Jekyll version of my blog
I was thinking about moving away from WordPress as blogging platform for quite some time but hesitated mainly because of the amount of work this would cause I was afraid of. The recent chaos on the...
I was thinking about moving away from WordPress as blogging platform for quite some time but hesitated mainly because of the amount of work this would cause I was afraid of. The recent chaos on the...
The book is split into three parts. The first one covers the basics of MVVM and .NET MAUI, while the second one goes hands on a full recipe app sample. The third part goes even deeper into specific...
My current side project is running on iOS and MacCatalyst. Even if both of them are able to share a lot of code, there are some challenges that you should be aware of if you’re going down this rout...
In my recent side project, TwistReader, I needed to modify the Border of an Entry to match my application’s primary color. The .NET MAUI Entry does not provide a direct way to change the color of t...
I took this course because I never did unit tests before. As unit testing became part of my daytime job, I needed a head start to unit testing. On researching resources about the topic of unit tes...
My current side project TimeTraverseHub has entered the stage where I need to add some global menus that are available everywhere in the app. Being a .NET MAUI app, I tried the naive approach first...
Leaving dormakaba AG The first two months of the year I spent with preparing my leaving of dormakaba AG at the end of February. As I made several templates and tools to use those templates for the...
As we have done the work for macOS, we can turn our attention now to the Windows operating system. Spoiler: this post will be far shorter than the last one. Recap Dealing with application windows...
As I posted already on my Mastodon feed, I recently had to deal with application windows for one of my side projects. It all started because I needed a secondary window of fixed size that can be op...
Announcements like this one are never easy, not for me and also not for others. In order to get stuff finished and done, I have to streamline my efforts when it comes to my side projects, though. ...