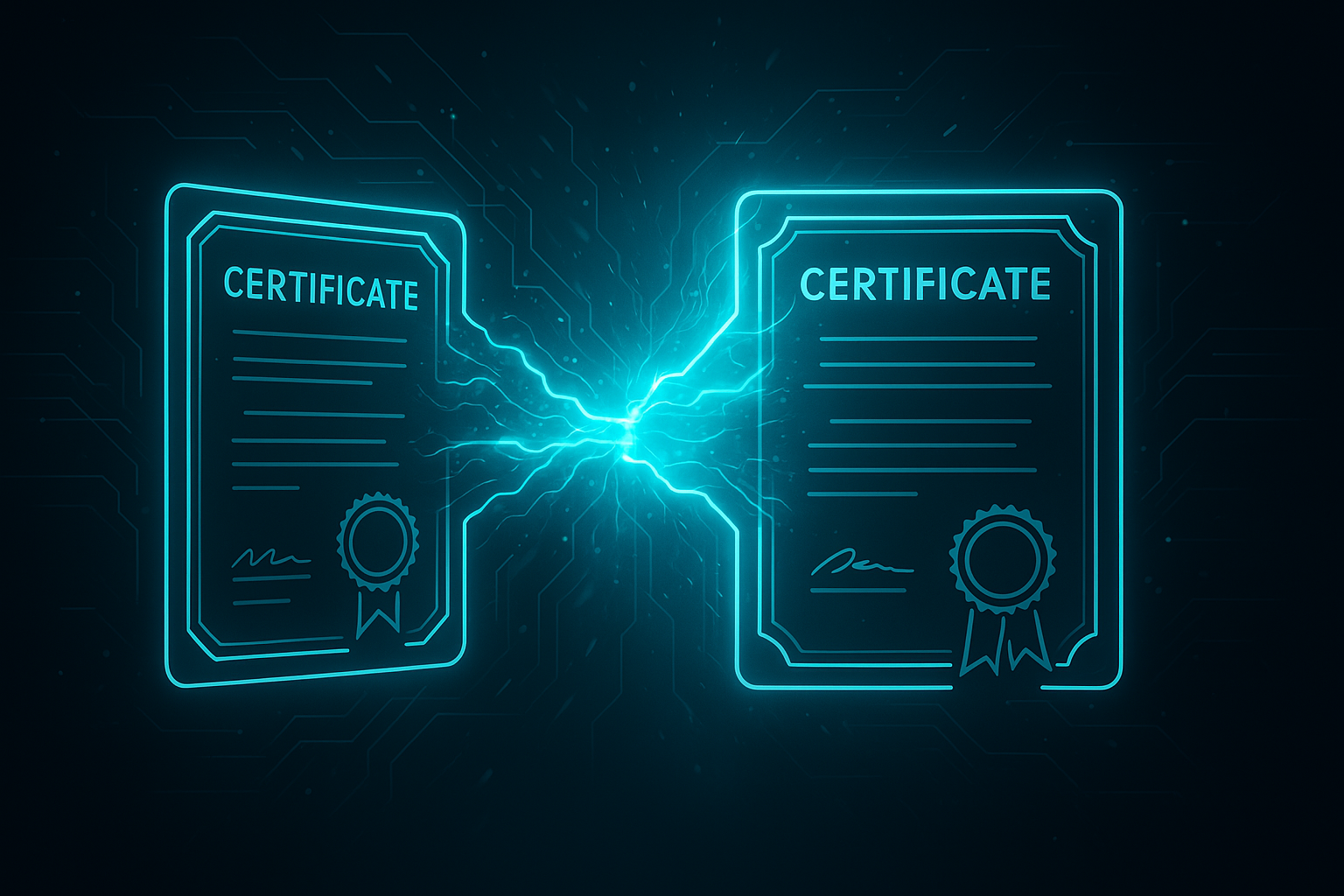
CI-Ready macOS Signing: Combining Apple Distribution & Installer Certificates for GitHub Actions
In my previous blog post, Exporting Apple Distribution Certificates for CI/CD — The Right Way, I shared a reliable method to extract and repackage Apple Distribution certificates into a clean .p12 ...